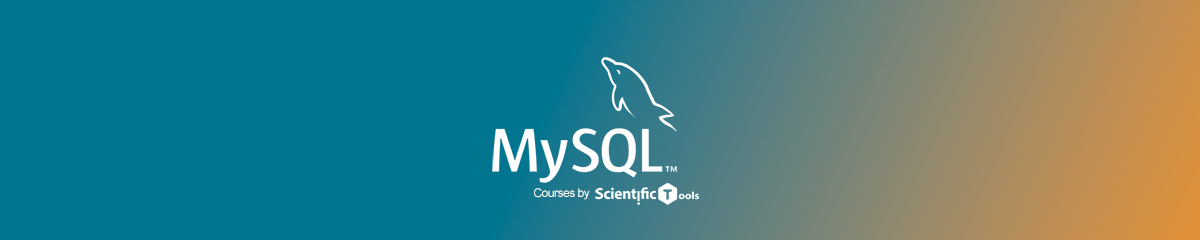
The MySQL OR operator is a logical operator used in SQL queries to combine two or more Boolean conditions. It is typically used in a WHERE clause to filter records based on multiple conditions. The OR operator returns TRUE if any of the conditions separated by OR is true.
Introduction to MySQL OR Operator
The OR operator in MySQL is used to check multiple conditions in a SQL query. It returns true (1) if any of the conditions being checked are true.
Basic Functioning of OR Operator
- True if Either is True: If at least one of the conditions is true (non-zero and not NULL), OR returns 1.
- False if Both are False: If both conditions are false (0), OR returns 0.
- NULL Handling: If one of the conditions is NULL and the other is false, OR returns NULL.
Examples of MySQL OR Operator
Let's take a books table with columns author, title, genre, and year_published for our examples.
Example 1: Basic OR Operations
Output Example:
Code
+------+--------+------+
| 1 OR 2 | 0 OR 0 | 0 OR 3 |
+------+--------+------+
| 1 | 0 | 1 |
+------+--------+------+
Example 2: OR with NULL Values
Output Example:
Code
+-----------+-----------+--------------+
| 1 OR NULL | 0 OR NULL | NULL OR NULL |
+-----------+-----------+--------------+
| 1 | NULL | NULL |
+-----------+-----------+--------------+
Practical Use of OR Operator in Queries
Example 3: Filtering Data with OR
To find books in either the 'Fiction' or 'Mystery' genre:
Output Example:
Code
+------------------+--------+
| title | genre |
+------------------+--------+
| Moonlight Shadow | Fiction|
| Secret Keeper | Mystery|
...
Example 4: Combining OR with AND
To find books published after 2010 in 'Fiction' or all 'Mystery' books:
SQL
SELECT title, genre, year_published
FROM books
WHERE (genre = 'Fiction' AND year_published > 2010) OR genre = 'Mystery';
Output Example:
Code
+------------------+--------+----------------+
| title | genre | year_published |
+------------------+--------+----------------+
| Moonlight Shadow | Fiction| 2012 |
| Secret Keeper | Mystery| 2009 |
...
Operator Precedence and Parentheses
- AND vs. OR Precedence: In SQL, AND has a higher precedence than OR.
- Changing Evaluation Order: Use parentheses to change the order in which conditions are evaluated.
Example 5: Precedence Demonstration
Output Example:
Summary
- The OR operator is used to check multiple conditions, where the result is true if any condition is true.
- It's important to understand how OR interacts with AND and how operator precedence affects the outcome of your queries.
- Parentheses can be used to explicitly define the order of evaluation in complex conditions.