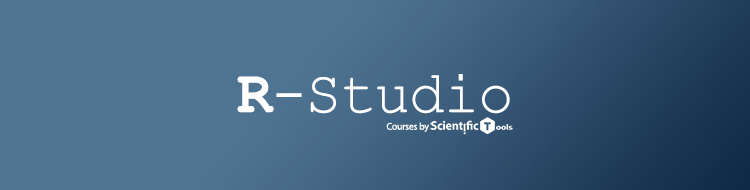
In RStudio, there are various methods available to import data and datasets into your R environment. You can use R basic function or use R packages.
Here is a detailed overview of the commonly used methods for importing data with examples:
Using Base R Functions:
a. read.table() and read.csv():
These functions are used to import data from plain text files with tabular or comma-separated values (CSV) format, respectively. They create a data frame in R containing the imported data.
Example:
# Import data from a tab-delimited text file
data <- read.table("data.txt", header = TRUE, sep = "\t")
# Import data from a CSV file
data <- read.csv("data.csv", header = TRUE)
b. read.delim() and read.csv2():
Similar to read.table() and read.csv(), these functions are used to import text files with tab-delimited or semicolon-separated values, respectively.
Example:
# Import data from a tab-delimited text file
data <- read.delim("data.txt", header = TRUE)
# Import data from a semicolon-separated file
data <- read.csv2("data.csv", header = TRUE)
Excel Files:
1- openxlsx Package:
To import data from an Excel file, you can use the read.xlsx() function from the openxlsx package. This function allows you to specify the file path, sheet name, and other optional parameters to read the data.
In the above example, data.xlsx is the name of the Excel file, and sheet = 1 indicates the first sheet in the Excel file. You can provide the appropriate sheet name or index to read data from a specific sheet.
2- readxl Package:
This package allows importing data from Microsoft Excel files (.xls and .xlsx formats). Functions like read_excel() can be used to import data from specific worksheets or ranges within Excel files.
Example:
# Import data from an Excel file
library(readxl)
data <- read_excel("data.xlsx", sheet = "Sheet1")
SPSS and SAS Files:
haven Package:
The haven package enables importing data from SPSS (.sav) and SAS (.sas7bdat) files. Functions like read_sav() and read_sas() can be used to read these file formats.
Example:
# Import data from an SPSS file
library(haven)
data <- read_sav("data.sav")
# Import data from a SAS file
data <- read_sas("data.sas7bdat")
Database Connections:
DBI Package:
The DBI package provides a standard interface for connecting to databases. You can establish connections to various databases such as MySQL, PostgreSQL, SQLite, etc. using functions like dbConnect(). Once connected, you can execute SQL queries and retrieve data into R.
Example:
# Import data from a MySQL database
library(DBI)
con <- dbConnect(RMySQL::MySQL(), dbname = "database", host = "localhost", port = 3306, user = "username", password = "password")
data <- dbGetQuery(con, "SELECT * FROM table")
dbDisconnect(con)
Web APIs:
httr Package:
The httr package provides functions to interact with web APIs and fetch data. Functions like GET() and POST() allow you to make HTTP requests and retrieve data in various formats, such as JSON or XML.
Example:
# Import data from a web API
library(httr)
response <- GET("https://api.example.com/data")
data <- content(response, "parsed")
Web Scraping:
rvest Package:
The rvest package enables web scraping by extracting data from HTML web pages. Functions like read_html() and html_nodes() help parse the HTML structure and extract desired data.
Example:
# Import data by web scraping
library(rvest)
webpage <- read_html("https://www.example.com")
data <- html_nodes(webpage, "selector")
Statistical Software Packages:
foreign Package:
The foreign package provides functions to import data files from various statistical software packages like Stata, Minitab, EViews, etc. Functions such as read.dta() and read.mtp() allow importing data from these formats.
Example:
# Import data from a Stata file
library(foreign)
data <- read.dta("data.dta")
# Import data from a Minitab file
data <- read.mtp("data.mtp")
Other File Formats:
readr Package:
The readr package provides a faster and more user-friendly alternative to the base R functions for reading delimited text files. Functions like read_csv() and read_tsv() offer improved performance and flexible options for importing data.
Example:
# Import data from a CSV file
library(readr)
data <- read_csv("data.csv")
# Import data from a tab-separated file
data <- read_tsv("data.tsv")
readbitmap Package:
This package allows importing image data from bitmap (BMP) files into R as arrays.
Example:
These methods offer flexibility in importing data into RStudio, allowing you to work with a variety of file formats, databases, web APIs, and statistical software packages. Choose the appropriate method based on your specific data source and format to ensure smooth data integration into your R projects.